- 16 May 2024
- 5 Minutes to read
- Print
- DarkLight
- PDF
Azure functions
- Updated on 16 May 2024
- 5 Minutes to read
- Print
- DarkLight
- PDF
.NET is a software framework developed by Microsoft that runs primarily on Microsoft Windows. It includes an extensive library of pre-written code and provides a runtime environment for executing code written in various programming languages. The framework is designed to provide a consistent object-oriented programming environment, whether code is executed on a server, browser, or desktop. It also includes a standard set of libraries for tasks such as connecting to databases, creating graphical user interfaces, and handling security.
Traceable provides a .NET agent to capture the requests inline. If, for some reason, Traceable cannot capture the request, the requests are passed through to the application. The following is a high-level deployment diagram:
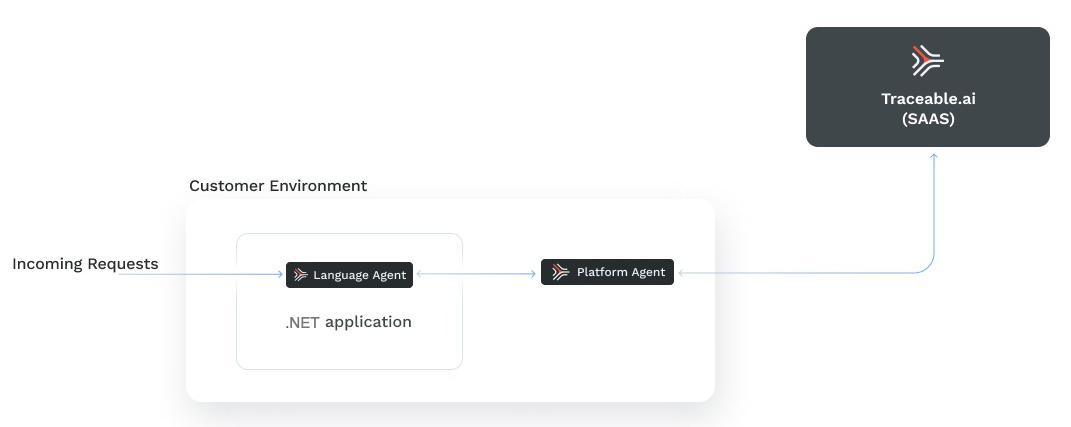
Before you begin
Make a note of the following before proceeding with Traceable’s .NET agent configuration:
Make sure that the Traceable Platform agent is already installed. For more installation information, see Platform agent.
Make a note of the Traceable Platform agent IP address. This would be used in configuration later.
Note that the Traceable Platform agent needs to be connected on non-TLS port 4317.
Ensure to allow the 4317 port as a firewall rule on the machine where the Traceable Platform agent is installed. For example, on a Windows machine’s internal firewall, you must allow inbound connections on the 4317 port.
Decide on a service name. This is required during configuration and is displayed in the Traceable Platform.
Traceable supports Azure Function with HTTP Trigger template v4 running on .NET 6 on .NET agent.
Supported framework
Traceable’s .NET agent supports Azure Functions. In the context of the Azure function, there are two different runtimes: In-process and isolated.
Traceable supports In-process runtime. At present, Traceable supports only HTTP trigger template based functions. The HTTP Trigger template is an Azure Functions template that allows you to create functions that respond to HTTP requests. When you create an Azure Function using the HTTP Trigger template, you create an endpoint that can be invoked via HTTP.
Configuration
Complete the following steps to configure Traceable’s .NET agent with Azure Functions:
Add the
Traceable.Agent
package to the project from NuGet gallery.dotnet add package Traceable.Agent
In the Azure portal, navigate to your project’s root directory and make the following code change to
startup.cs
file. In Azure Functions projects, thestartup.cs
file is a crucial component for configuring the application's services and request processing pipeline. It's typically located in the project's root directory.using Microsoft.AspNetCore.Server.Kestrel; using Microsoft.Azure.Functions.Extensions.DependencyInjection; using Microsoft.Extensions.DependencyInjection; using Traceable; //<- additional change for Traceable [assembly: FunctionsStartup(typeof(Company.Function.Startup))] namespace Company.Function { public class Startup : FunctionsStartup { public override void Configure(IFunctionsHostBuilder builder) { // changes for traceable begin var traceableAgent = TraceableAgentBuilder.CreateBuilder() .AddInstrumentation(Instrumentations.AzureFunctionsInProcess) .Build(); builder.Services.AddSingleton(traceableAgent); // changes for traceable end builder.Services.AddLogging(); } } }
Make a note of the code that you need to insert:
// changes for traceable begin var traceableAgent = TraceableAgentBuilder.CreateBuilder() .AddInstrumentation(Instrumentations.AzureFunctionsInProcess) .Build(); builder.Services.AddSingleton(traceableAgent); // changes for traceable end
Note
If you are not using the above
class
, you can create one by referring to Microsoft documentation.Add the following environment variables for configuration. In your Azure function application, navigate to Settings → Configuration to add the environment variable and save the configuration.
Environment variable
Description
TA_SERVICE_NAME
(Optional) The name with which you would like to identify the service in Traceable Platform. The default service name is
dotnetagent
when no service name is assigned.TA_REPORTING_ENDPOINT
The endpoint for reporting the traces. This is a mandatory field.
OTLP - For OTLP reporter, use http://<traceable_platform_agent_host>:4317
Note
HTTPS communication is currently not supported.
TA_DATA_CAPTURE_HTTP_HEADERS_REQUEST
(Optional) When set to
false
, the agent disables request capturing.TA_DATA_CAPTURE_HTTP_HEADERS_RESPONSE
(Optional) When set to
false
, the agent disables response capturing.TA_DATA_CAPTURE_HTTP_BODY_REQUEST
(Optional) When set to
false
, the agent disables request body capturing.TA_DATA_CAPTURE_HTTP_BODY_RESPONSE
(Optional) When set to
false
, the agent disables response body capturing.TA_DATA_CAPTURE_BODY_MAX_SIZE_BYTES
(Optional) Configure the maximum size of the body to capture. The default value is 128 KiB.
TA_DATA_CAPTURE_ALLOWED_CONTENT_TYPES
(Optional) Comma-separated values of content type to capture. The default is [json, x-www-form-urlencoded]. For example, [json] will record any request body with a content-type header that includes “json”.
TA_ENABLED
(Optional) Set it to
false
, if you wish to disable Traceable’s .NET agent.TA_TELEMETRY_STARTUP_SPAN_ENABLED
(Optional) When set to
true
, an internal span is created and exported when the agent is initialized and started.Deploy the application to Azure.
Verification
Send data to your application. On successful configuration, you will see Span data in the Traceable Platform.
.png)
Upgrade
Complete the following steps to upgrade the package:
Enter the following command to upgrade:
dotnet add package Traceable.Agent
Deploy the application to Azure.
Uninstall
Complete the following steps to uninstall the .NET agent:
Remove the .NET agent bootstrap code from
startup.cs
file.Remove the Traceable.Agent package dependency from the project
csproj
file. Following is an example ofcsproj
file. Remove<PackageReference Include="Traceable.Agent" Version="1.0.0-rc.5" />
fromcsproj
file.<Project Sdk="Microsoft.NET.Sdk"> <PropertyGroup> <TargetFramework>net6.0</TargetFramework> <AzureFunctionsVersion>v4</AzureFunctionsVersion> <RootNamespace>azure_app_inprocess</RootNamespace> </PropertyGroup> <ItemGroup> <PackageReference Include="Microsoft.AspNetCore.Hosting" Version="2.2.7" /> <PackageReference Include="Microsoft.AspNetCore.Http" Version="2.2.2" /> <PackageReference Include="Microsoft.AspNetCore.Http.Features" Version="5.0.17" /> <PackageReference Include="Microsoft.Azure.Functions.Extensions" Version="1.1.0" /> <PackageReference Include="Microsoft.Azure.WebJobs" Version="3.0.39" /> <PackageReference Include="Microsoft.Azure.WebJobs.Extensions.EventHubs" Version="6.0.2" /> <PackageReference Include="Microsoft.NET.Sdk.Functions" Version="4.2.0" /> <PackageReference Include="System.Reactive.Core" Version="6.0.0" /> <PackageReference Include="Microsoft.AspNetCore.Http.Extensions" Version="2.2.0" /> <PackageReference Include="Microsoft.IO.RecyclableMemoryStream" Version="3.0.0" /> <PackageReference Include="Microsoft.AspNetCore.Http.Features" Version="5.0.17" /> <PackageReference Include="Traceable.Agent" Version="1.0.0-rc.5" /> </ItemGroup> <ItemGroup> <None Update="host.json"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="local.settings.json"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> <CopyToPublishDirectory>Never</CopyToPublishDirectory> </None> </ItemGroup> </Project>
Perform a dotnet restore.
dotnet restore
Deploy the application to Azure.